1、组件回调函数中,需要传自定义参数时,可以传arguments或者$event作为回调的参数,后面加上自定义参数。
注:传arguments在app端取不到value值,h5可以取到,所以最终传$event。
vue:
@change="changeFun(arguments,item)"
@change="changeFun($event,item)"
js:
changeFun(value,item){
//value为回调的参数,item为自定义参数
}
2、vue中最好不要写比较复杂的js,最好不要写js,语法类似小程序,小程序不支持html有js语法及函数,打包成app会报错,会显示undefined。
例如:.substring();格式化时间;
解决方案:可以用计算属性代替html中的计算之类的
3、uni.showModal ,app上 content参数为空值,弹窗不显示,但是h5会显示,title可为空。
4、选择图片,需要限制大小,否则上传返回的res.data为空
uni.chooseImage({
count: 9, //默认9
sizeType: ['original', 'compressed'], //可以指定是原图还是压缩图,默认二者都有
sourceType: ['album'], //从相册选择
success: function(res) {
let tempFilePaths = [];
res.tempFiles.forEach(item => {
if (item.size < 1048576) {
tempFilePaths.push(item.path);
}
})
if (tempFilePaths.length !== res.tempFiles.length) {
self.$showToast({
text: '请选择1M内的图片'
})
}
if (tempFilePaths.length > 0) {
uni.setStorageSync('activity_products', '');
uni.setStorageSync('activity_photos', tempFilePaths);
uni.navigateTo({
url: './issue1'
})
}
}
});
uni.uploadFile({
url: apiList.host + '/gateway',
filePath: item,
name: 'file',
header: {
'content-type': 'multipart/form-data', // 文件类型
'method': 'file.upload.direct',
'mall_code': self.$store.state.mall_code,
'_app-key': 'MjIyMg==',
'_authorization': self.$store.state.token || '',
},
success: function(res) {
console.log('res.data', res.data)
if (res.data) {
let data = JSON.parse(res.data);
if (data.code == 200) {
let url = data.data;
photos.push(url);
if (photos.length == self.activity_photos.length) {
self.params.photos = photos.join(',');
self.post();
}
} else {
self.$showToast({
text: data.error
})
uni.hideLoading();
}
} else {
self.$showToast({
text: '发布失败'
})
uni.hideLoading();
}
},
fail: function(res) {
self.$showToast({
text: res.msg
});
uni.hideLoading();
},
})
5、app中 使用store,需要在main.js中加 Vue.prototype.$store = store;
6、app中 给变量赋值,data中必须要有此变量,否则报错。
7、app运行报错:uni-app unexpected character "
原因:页面中的class=" 之后接的数据换行了
8、app运行报错: [错误] ./pages/member/mobile_modify/mobile_modify.wxml:1:2067: Bad attr data-event-opts
with message: unexpected token email
.
解决:input标签中写了 v-model="a==1?a:b",这种写法不支持
9、app运行报错:TypeError: $gwx is not a function
网上方法1(无效):是那个美元符号的问题。。加个空格
解决:是语法不兼容有问题就会报这个错,至于哪里不兼容需要自己找
1.组件中的props 不能使用vue原型的全局数据,需要单独引入使用
2.localStorage.getItem()在app中不识别,不能使用,需用uni的自定义api
3.组件内不能使用data作为props
4.data()中定义的变量,若语法有问题,那么onLoad()中打印任何data中的变量,都为undefined
5.vue中使用函数@tap="autFun('btns','editStock','fun',()=>{return editStockShow(item)})",报错bad attr 'data-ecent-opts' with message:unexpected
10、如下图
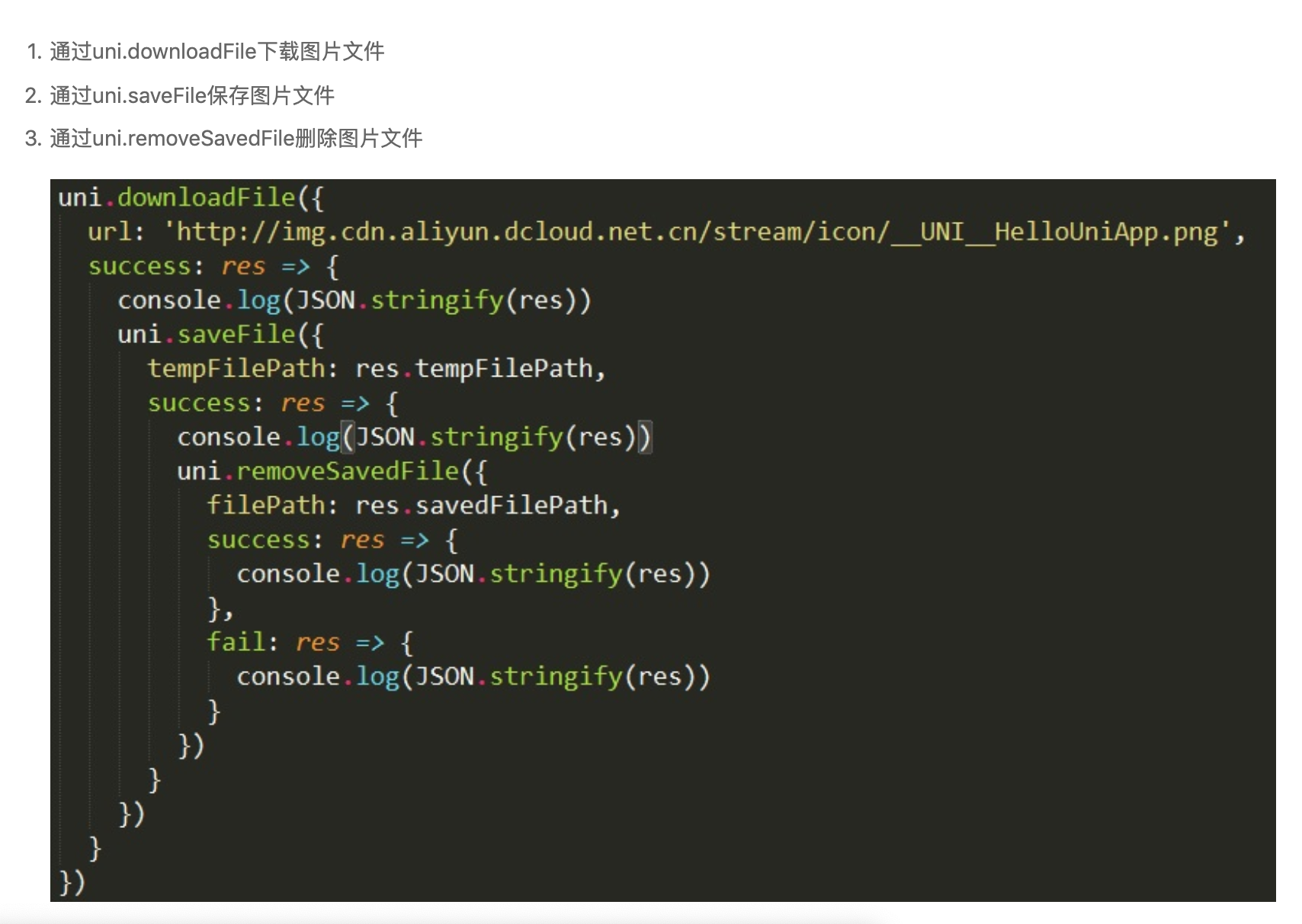
11、在app端取不到value值,h5可以取到
解决:
将arguments,改为$event
12、在app端会报错。.title为undefined,h5不会
解决:
改为计算属性
13、html中最好不要做太多js操作,例如格式化时间,app中会显示undefined
14、保存图片到相册 app
1、安卓无效
//利用plus完成保存到相册
let filename = Math.random() +".png"
plus.downloader.createDownload(this.imageURL, {
filename:"_downloads/"+filename
}, (download,status)=>{
if(status==200){//下载成功
plus.gallery.save( download.filename, ()=>{
uni.hideLoading();
uni.showToast({
title: '保存成功!'
});
})
}
}).start()
2、ios有效 安卓无效,保存失败
uni.downloadFile({
url: this.code,
success(res) {
uni.saveImageToPhotosAlbum({
filePath: res.tempFilePath,
success: function() {
self.$showToast({
text: '保存成功'
})
},
fail: function(err) {
console.log(err)
self.$showToast({
text: '保存失败,请截图分享'
})
}
});
},
fail: function(err) {
console.log(err)
self.$showToast({
text: '下载失败,请截图分享'
})
}
})
3、canvas绘图 保存到本地 ios有效 安卓 h5绘图不出来,可能是因为下载的图片是base64的;drawImg()如果是base64图片可以直接canvas绘图,不用downloadfile了,否则安卓不显示;base64直接绘图,h5不显示,安卓ios显示
<canvas style="width: 300px; height: 300px;margin:0 auto" canvas-id="myCanvas" ></canvas>
uni.downloadFile({
url: res.data,
success(res) {
self.drawImg(res.tempFilePath);
},
fail: function(err) {
console.log(err)
self.$showToast({
text: '下载失败'
})
}
})
drawImg(code) {
setTimeout(() => {
const ctx = uni.createCanvasContext('myCanvas');
ctx.drawImage(code, 0, 0, 300, 300);
ctx.setFontSize(14);
ctx.setFillStyle('#6D7278');
ctx.fillText('长按或扫描二维码访问我的门店', 50, 280);
ctx.draw();
this.code = true;
}, 500);
},
save() {
let self = this;
uni.canvasToTempFilePath({
x: 0,
y: 0,
width: 300,
height: 300,
destWidth: 600,
destHeight: 600,
canvasId: 'myCanvas',
success: function(res) {
console.log(res.tempFilePath)
uni.saveImageToPhotosAlbum({
filePath: res.tempFilePath,
success: function() {
self.$showToast({
text: '保存成功'
})
},
fail: function(err) {
console.log(err)
self.$showToast({
text: '保存失败,请截图分享'
})
}
});
}
})
},
15、app中,组件props为string类型,传值为空串“”,app会显示为true,若默认default为“”,其子组件的props也会显示为true
16、复制api
self.copyText = function(text) {
uni.setClipboardData({
data: text,
success: function(res) {
uni.getClipboardData({
success: function(res) {
// app会有默认提示“内容已复制”
// self.$common.Toast({
// text: self.i18.order.copySuc
// });
}
})
}
})
}
解决有默认提示
17、uni.uploadFile() h5 不能写content-type参数,写了会报错,app也可以不写
18、picker:有一个app兼容问题,如果默认值为空,首次手动赋值的时候如果index是0, 页面显示不更新,我看了下文档估计是组件默认值为0的原因,H5没有,我现在处理的是index为0的话就先改为其他值,然后setTimeout赋正确值
19、页面隐藏滚动条,包括横向滚动条
//1.设置全局样式,此方法无效
::-webkit-scrollbar {
width: 0;
height: 0;
color: transparent;
}
//2.设置全局样式,此方法h5页面有效,app无效
::-webkit-scrollbar {
display: none;
}
//3.page.json 页面设置此参数,仅对app有效
{
"path": "pages/home/classifyDetail/classifyDetail",
"style": {
"navigationBarTitleText": "分类详情",
"navigationBarBackgroundColor": "#FFFFFF",
"app-plus": {
// 禁用原生导航栏
"titleNView": false,
// 手机端隐藏滚动条,保留滚动效果
"scrollIndicator":"none"
}
}
},
20、解决安卓点击两次返回按钮关闭应用问题
// 重写quit方法改为隐藏至后台
if (!this.$common.isIOS()) {
let main = plus.android.runtimeMainActivity();
plus.runtime.quit = function () {
main.moveTaskToBack(false);
}
}
21、跳转最好都写绝对路径,/pages/……
遇过的问题:h5中,首页写相对路径,./ ../ 首次进页面跳转成功,进入其他tab页面,再回来跳转,无效了
@tap=navigateTo(url)
navigator标签
uni.navigateTo()