<template>
<view class="container">
<map id="map" @regionchange="changeMap" @updated="onMapFinish" class="map_layout" :latitude="latitude" :longitude="longitude" :markers="markers" @markertap="markertapClick" scale='14'></map>
<scroll-view class="content_layout u-flex-col" scroll-y="true" v-if="dataItem != null">
<view class="item_layout u-flex-col">
<text style="font-size: 36rpx;font-weight: bold;color: #16181A;">{{dataItem.name}}</text>
<text style="font-size: 28rpx;font-weight: 400;color: #575F66;margin-top: 10rpx;">{{(dataItem.distance/1000).toFixed(2)}}公里 | {{dataItem.address}}</text>
</view>
<view class="item_layout u-flex-col" style="margin-top: 20rpx;">
<text style="font-size: 36rpx;font-weight: bold;color: #16181A;">停车场概况</text>
<view class="u-flex u-flex-align-items u-flex-justify-content-space-between" style="margin-top: 24rpx;">
<view class="u-flex-col u-flex-align-items u-flex-justify-content">
<text style="font-size: 40rpx;font-weight: bold;color: #1890FF;">{{dataItem.num}}</text>
<text style="font-size: 24rpx;font-weight: 400;color: #575F66;margin-top: 8rpx;">总车位数</text>
</view>
<view class="u-flex-col u-flex-align-items u-flex-justify-content">
<text style="font-size: 40rpx;font-weight: bold;color: #1890FF;">{{dataItem.leftnum}}</text>
<text style="font-size: 24rpx;font-weight: 400;color: #575F66;margin-top: 8rpx;">剩余车位</text>
</view>
<view class="u-flex-col u-flex-align-items u-flex-justify-content">
<text style="font-size: 40rpx;font-weight: bold;color: #1890FF;">{{dataItem.price}}</text>
<text style="font-size: 24rpx;font-weight: 400;color: #575F66;margin-top: 8rpx;">{{dataItem.priceunit}}</text>
</view>
</view>
</view>
<view class="item_layout u-flex-col" style="margin-top: 20rpx;">
<text style="font-size: 36rpx;font-weight: bold;color: #16181A;">收费描述</text>
<text style="font-size: 28rpx;font-weight: 400;color: #575F66;margin-top: 20rpx;">{{dataItem.pricedesc}}</text>
</view>
</scroll-view>
</view>
</template>
<script>
import gcoord from '@/model/gcoord.js'
import {CommonModel} from '@/model/common.js'
const commonModel = new CommonModel()
export default {
data() {
return {
title: 'map',
latitude: 0,
longitude: 0,
markers: [],
pressedMarker:null,
dataList:[],
dataItem:null,
}
},
onLoad() {
//this.getMyLocation()
},
methods: {
toJSON(){},
onMapFinish: function() {
console.log('现在可以开始监听@regionchange了');
},
changeMap(e){
let type = '';
const { platform } = uni.getSystemInfoSync();
console.log(platform)
switch (platform) {
case 'android':
type = e.type;
break;
case 'ios':
type = e.detail.type;
break;
default:
type = e.type;
break;
}
console.log("type:"+type);
if (type === 'end') {
// 处理拖拽结束时的逻辑
console.log("结束了");
let mapCtx =uni.createMapContext('map',this);
mapCtx.getCenterLocation({
type: 'gcj02',
success:res=> {
// 当前中心经纬度位置
if(this.latitude != res.latitude || this.longitude !=res.longitude){
console.log("和上次不一样")
this.latitude = res.latitude;
this.longitude =res.longitude;
let result = gcoord.transform(
[this.longitude, this.latitude], // 经纬度坐标
gcoord.GCJ02, // 当前坐标系
gcoord.BD09 // 目标坐标系
);
this.getNearbyParkingList(result[0],result[1]);
}
}
})
}
},
markertapClick(e){
console.log(e)
//点击非当前位置标记时,即停车场marker
if(e.detail.markerId != 0){
let index = -1;
let item = null;
if(this.pressedMarker != null){
item = this.pressedMarker;
index = this.pressedMarker.id;
item.width = 42;
item.height = 46;
item.iconPath = '/static/images/icon_parking_normal.png';
//this.$set(this.markers,index,item);//正确方式
}
index = e.detail.markerId;
item = this.markers[index];
item.width = 63;
item.height = 69;
item.iconPath = '/static/images/icon_parking_pressed.png';
//this.$set(this.markers,index,item);//正确方式
this.pressedMarker = item;
this.dataItem = this.dataList[index-1];
}
},
getMyLocation () {
uni.getLocation({
// map组件默认为国测局坐标gcj02,调用 uni.getLocation返回结果传递给组件时,需指定 type 为 gcj02
type: 'gcj02',
success: ({ longitude, latitude }) => {
// 定位得到的经纬度
console.log('当前位置的经度:' + longitude)
console.log('当前位置的纬度:' + latitude)
this.longitude = longitude
this.latitude = latitude
let result = gcoord.transform(
[this.longitude, this.latitude], // 经纬度坐标
gcoord.GCJ02, // 当前坐标系
gcoord.BD09 // 目标坐标系
);
this.getNearbyParkingList(result[0],result[1]);
}
})
},
getNearbyParkingList(longitude,latitude){
let that = this;
let para = {
lat:latitude,
lng:longitude,
}
commonModel.getNearbyParkingList(para).then(res => {
if(res.data){
that.markers = [];
that.markers.push({
id:0,
longitude:that.longitude,
latitude:that.latitude,
iconPath: '/static/images/icon_map_location.png',
width:24,
height:35,
});
that.dataList = res.data.list;
for(var i=0;i<that.dataList.length;i++){
let child = that.dataList[i];
let gc = gcoord.transform(
[child.lng, child.lat], // 经纬度坐标
gcoord.BD09, // 当前坐标系
gcoord.GCJ02 // 目标坐标系
);
if(i == 0){
that.dataItem = child;
that.pressedMarker = {
id:(i+1),
longitude:gc[0],
latitude:gc[1],
iconPath: '/static/images/icon_parking_pressed.png',
width:63,
height:69,
}
that.markers.push(that.pressedMarker);
}else{
that.markers.push({
id:(i+1),
longitude:gc[0],
latitude:gc[1],
iconPath: '/static/images/icon_parking_normal.png',
width:42,
height:46,
})
}
}
}else{
uni.showToast({
title: res.msg,
icon: 'none'
})
}
})
},
}
}
</script>
<style>
.item_layout{
background-color: #fff;
padding: 24rpx;
}
.content_layout{
width: 100%;
height: 50vh;
}
.map_layout{
width: 100%;
height: 50vh;
}
.container{
width: 100%;
min-height: 100%;
background-color: #F5F7FA;
box-sizing: border-box;
}
</style>

8***@qq.com
- 发布:2023-06-10 13:41
- 更新:2023-06-10 13:41
- 阅读:579
uniapp在支付宝小程序上无法显示地图,在微信小程序可以正常显示
分类:uni-app
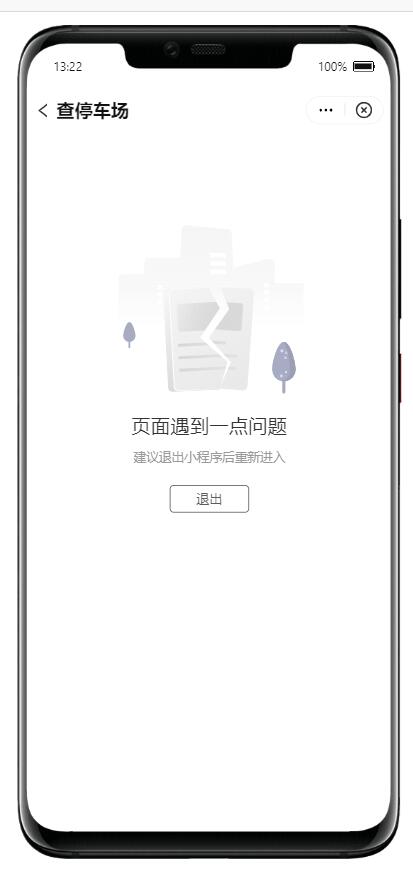
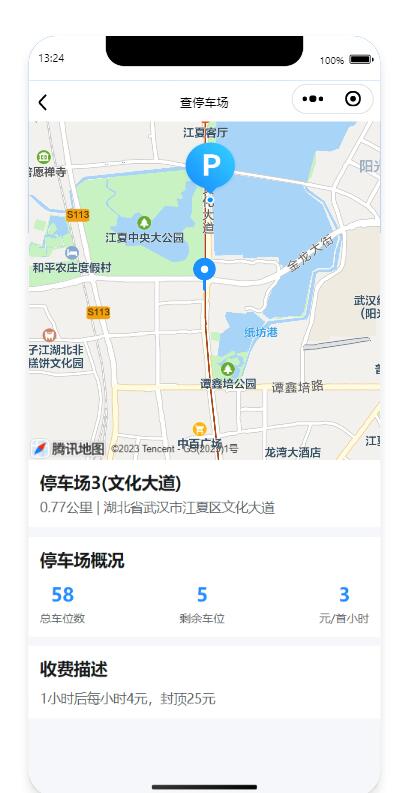